How to Open JSON Files
Grady Johnson · October 17th, 2023 · Last Updated: December 6th, 2023Whether you're a developer, data analyst, or just someone who wants to access information stored in a JSON file, knowing how to open JSON files is essential. In this comprehensive guide, we will explore various methods and tools for opening JSON files, regardless of your technical background.
What is JSON?
JSON, short for JavaScript Object Notation, is a lightweight data-interchange format that was invented in the early 2000s by Douglas Crockford. While it was originally invented to transmit data between a web browser and a web server, today it is also used as a format for configuration and data files. Unlike other data-interchange formats such as XML, JSON is incredibly easy for humans to read and write which has largely contributed to its rise in popularity over the last couple of decades.
JSON data consists of key-value pairs, where keys are strings enclosed in double quotes, followed by a colon, and then a value. Values can be strings, numbers, objects, arrays, booleans, or null. Here's a simple example of JSON data:
{
"name": "John Doe",
"age": 30,
"isStudent": false,
"hobbies": [
"reading",
"traveling"
]
}
Understanding JSON
JSON data can be stored in files with a .json
extension. These files can range from small configuration files of just a few lines to large datasets comprised of hundreds or even thousands of lines. It's important to understand how JSON data is structured before attempting to open JSON files. JSON files are typically well-organized, have a hierarchical structure, and are comprised of the following data types:
Objects: JSON data is enclosed in curly braces
{}
and consists of key-value pairs.json{ "title": "JSON Objects", "description": "This entire snippet is an example of a JSON object." }
Arrays: Values in JSON can be arrays, denoted by square brackets
[]
. Arrays can contains primitives such as strings or numbers or they can contain objects.json["tiger","lion","cheetah"]
Strings: Textual data is enclosed in double quotes.
json"This is an example of a string"
Numbers: Numeric values can be integers or floating-point numbers.
json5 3.14
Booleans: True or false values are represented as true or false.
jsontrue false
Null: The absence of a value is represented as null.
jsonnull
Methods for Opening JSON Files
Opening a JSON file can be done using various methods, depending on your needs and technical proficiency.
Using Text Editors
If you simply want to view the contents of a JSON file, a basic text editor will suffice. Common text editors like Notepad (Windows), TextEdit (macOS), or any code editor like Visual Studio Code or Sublime Text can be used.
- Open the text editor.
- Navigate to "File" > "Open" and select your JSON file.
- The JSON data will be displayed in the editor, and you can read or make minor edits. However, for more advanced tasks like parsing and manipulating JSON data, you'll need additional tools.
Using Command Line Tools
For those comfortable with the command line, several command-line tools can help you open and work with JSON files. These tools are available on most operating systems.
jq (Linux/macOS/Windows): jq is a powerful command-line JSON processor that allows you to filter, transform, and manipulate JSON data easily. You can install it via package managers like apt or brew, or download it from the official website. Example usage:
shelljq '.name' data.json
cat (Linux/macOS) or type (Windows): You can also use basic command-line utilities like
cat
ortype
to display the content of a JSON file directly in the terminal. Example usage:shellcat data.json
Using Programming Languages
To work with JSON files programmatically and perform more complex operations, you can use programming languages such as JavaScript, Python, or Ruby. Most modern programming languages provide libraries and modules for parsing and manipulating JSON data.
Using Online Tools
If you don't want to download a program just to view a JSON file (or simply can't because of your company's IT policies), you can use online tools such as betterjson.com to upload a JSON file and view its contents. betterjson.com can also format your JSON if it's been compacted into one line, a process known as "minification".
To open a JSON file with betterjson.com, simply click the upload button in the editor and then navigate to the location of the JSON file on your computer.
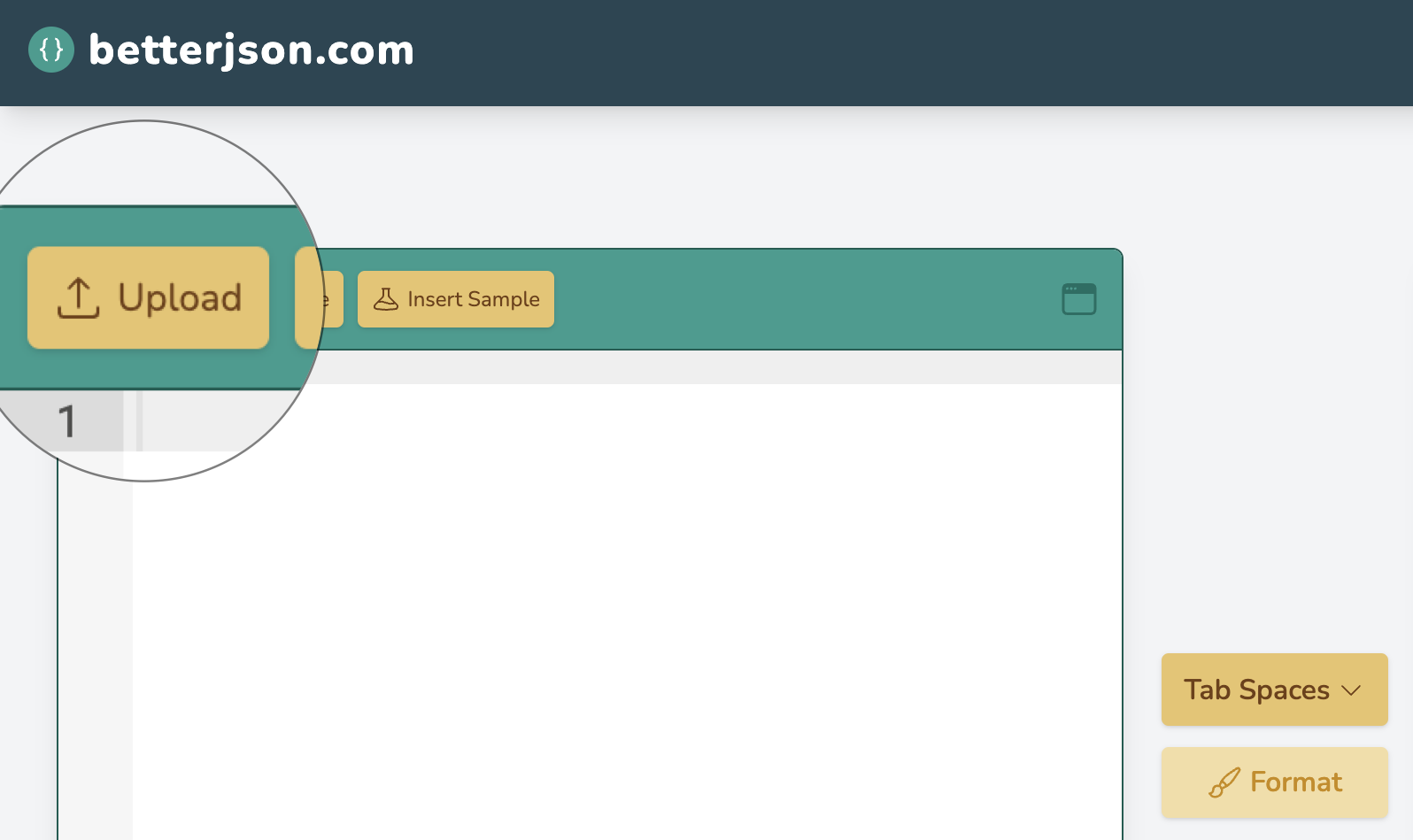
Viewing JSON Data
JSON data is hierarchical, and you can navigate its structure to access specific elements. Keys are used to access values within objects, and indexes are used to access elements within arrays. For instance, in the following JSON data:
{
"name": "John Doe",
"age": 30,
"isStudent": false,
"hobbies": [
"reading",
"traveling"
]
}
To access the "name" value, you would use the key "name". To access the second hobby ("traveling"), you would use the key "hobbies" followed by the index [1].
Searching JSON Data
If the JSON file is extensive, searching for specific data can be challenging. However, many text editors and online JSON viewers offer search functionality, allowing you to quickly locate the information you need.
In text editors:
- Use the keyboard shortcut (e.g.,
Ctrl+F
on Windows orCommand+F
on macOS) to open the search bar. - Enter your search query, and the editor will highlight matching results.
In online JSON viewers:
- Look for a search or find feature provided by the viewer.
- Enter your search query, and the viewer will locate and highlight matching data within the JSON structure.
Searching JSON Data on betterjson.com
There are two ways you can search JSON files using betterjson.com.
Search box: Like most text editors, betterjson.com supports searching files via a search box. The search box can be launched using the find keyboard shortcut (
Ctrl+F
on Windows orCommand+F
on macOS). When you use the find keyboard shortcut in either the JSON editor or JSON viewer on betterjson.com, a search box will appear in the upper right-hand corner as shown below.An example of searching JSON data on betterjson.com. Filter box: betterjson.com supports searching/filtering JSON data using JSONPath. With the right JSONPath expression, you can search for JSON objects that match certain criteria. Take the example JSON file below that models a store that sells books:
json{ "store": { "books": [ { "category": "reference", "author": "Nigel Rees", "title": "Sayings of the Century", "price": 8.95 }, { "category": "fiction", "author": "Evelyn Waugh", "title": "Sword of Honour", "price": 12.99 }, { "category": "fiction", "author": "Herman Melville", "title": "Moby Dick", "isbn": "0-553-21311-3", "price": 8.99 }, { "category": "fiction", "author": "J. R. R. Tolkien", "title": "The Lord of the Rings", "isbn": "0-395-19395-8", "price": 22.99 } ] } }
To search for and filter books less than $10, you could enter the following JSONPath expression:
$.store.books[?(@.price<10)]
An example of searching/filtering JSON data using a JSONPath expression on betterjson.com. Any JSON data that doesn't match the JSONPath expression will be temporarily hidden from the JSON viewer until the expression is cleared from the filter box.
Interested in learning more about JSONPath? Check out our article on JSONPath titled How to Query JSON Using JSONPath.
Conclusion
In recent years, JSON has become the preeminent data-interchange format and is employed in variety of contexts, from web development to configuration and data storage. By understanding the methods outlined in this guide, you can efficiently open JSON files, view their contents, and even perform basic operations without needing to learn a programming language.
Whether you prefer using text editors, online JSON viewers, or command line tools, the ability to access and work with JSON files will undoubtedly prove beneficial in many aspects of your digital life. So, pick the method that best suits your needs and embark on your journey to master the world of JSON data.